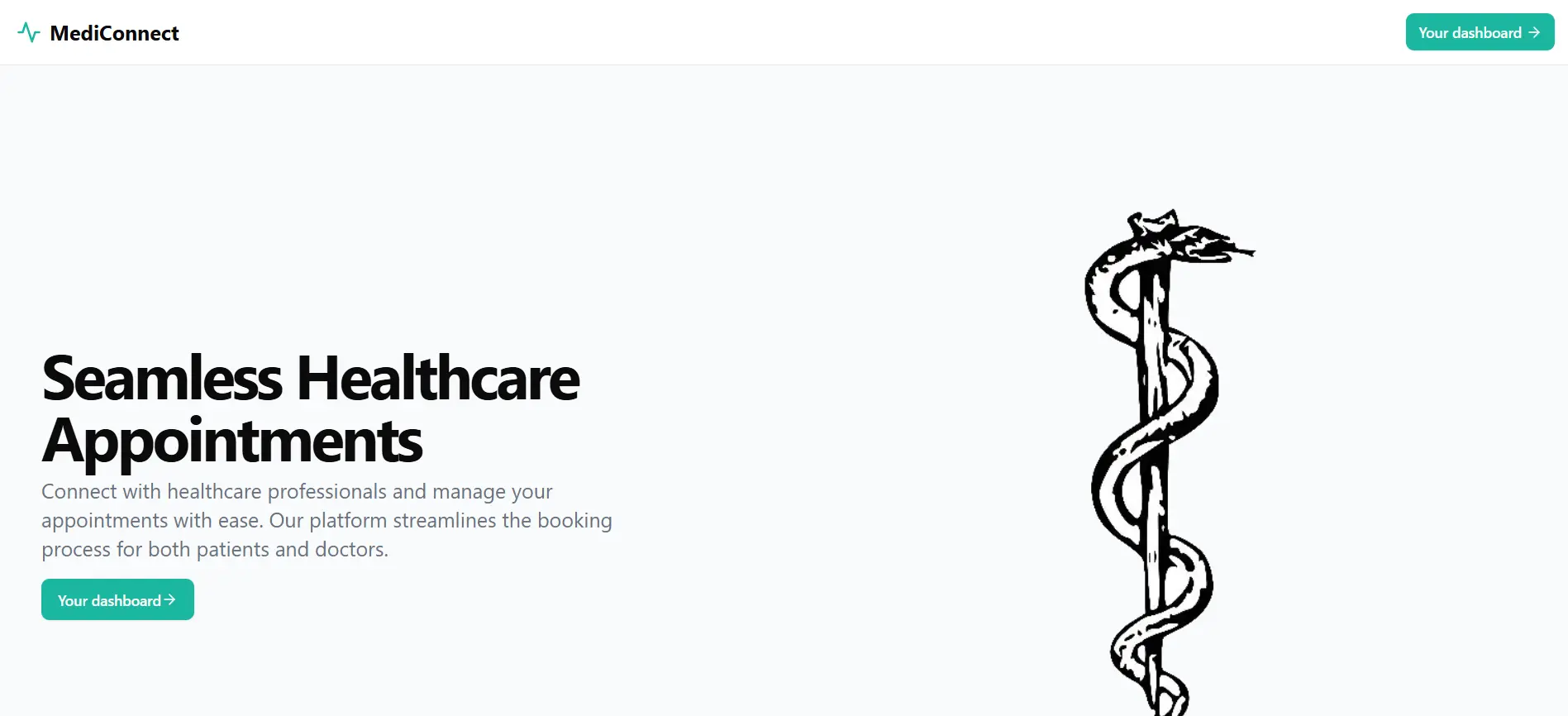
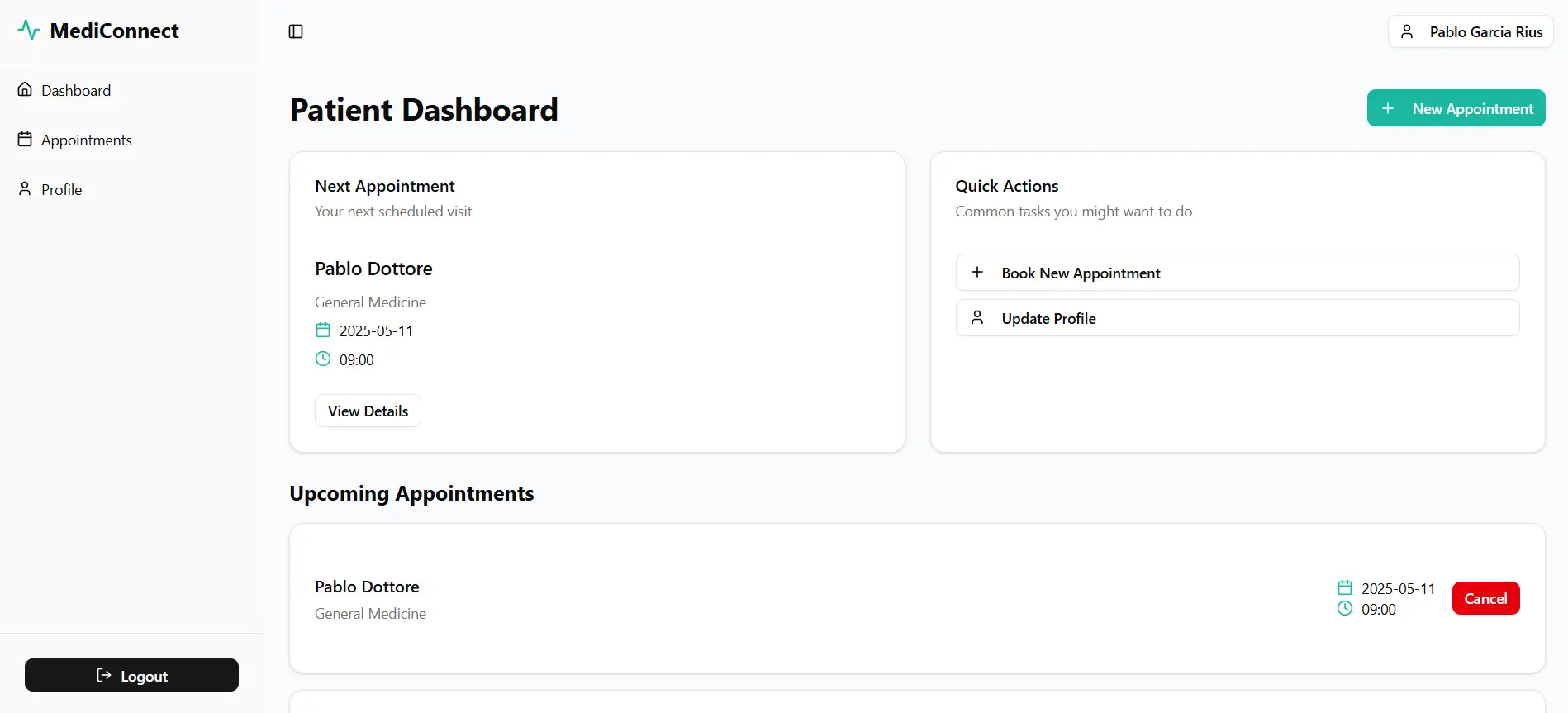
Project Overview
MediConnect is an online healthcare platform that bridges the gap between patients and healthcare providers. Built using the T3 stack (Next.js, TypeScript, and Prisma), this platform offers an intuitive interface for patients to book appointments and for doctors to manage their schedules efficiently.
This project represents my first implementation of the T3 stack, combining the power of Next.js for frontend and API routes, TypeScript for type safety, and Prisma for database operations. The platform also incorporates OAuth authentication with the Prisma adapter to ensure secure user sessions.
Technical Highlights
Dual Role System
Separate interfaces and permissions for patients and doctors, with role-specific features.
Appointment Management
Intuitive booking system for patients and schedule management for doctors.
Secure Authentication
OAuth integration with secure credentials and secure session management using Prisma adapter.
Prisma ORM
Type-safe database operations with Prisma for efficient data management.
Technical Implementation
MediConnect leverages the T3 stack to create a robust and type-safe application. Here's a breakdown of the key technical aspects:
- Next.js: Provides server-side rendering, API routes, and optimized performance for a seamless user experience.
- TypeScript: Ensures type safety throughout the application, reducing runtime errors and improving developer experience.
- Prisma: Offers a type-safe database client for efficient data modeling and querying, simplifying database operations.
- OAuth Authentication: Implements secure user authentication with multiple providers (Google, GitHub) using the Prisma adapter.
- Tailwind CSS: Provides utility-first styling for a responsive and consistent UI across all devices.
Quality Assurance Process
MediConnect was developed with a strong focus on quality assurance. A comprehensive testing plan was implemented to ensure the platform's reliability and security:
Challenges and Solutions
Developing MediConnect presented several challenges that required creative solutions:
- Dual Role System: Implementing different views and permissions for patients and doctors required careful planning of the database schema and authentication flow. I solved this by creating a role field in the User model and implementing role-based access control throughout the application.
- Appointment Scheduling Logic: Creating a system that prevents double-bookings and handles time slots efficiently was complex. I implemented a custom scheduling algorithm that checks for conflicts and validates availability before confirming appointments.
- Type Safety with Prisma: Ensuring type safety across the application while working with Prisma required careful setup of TypeScript types and interfaces. I created utility types that extend Prisma's generated types to maintain consistency throughout the codebase.
- OAuth Integration: Configuring OAuth providers with the Prisma adapter required precise implementation to ensure secure authentication. I followed best practices for environment variable management and session handling to maintain security.
Lessons Learned
MediConnect was my first project using the T3 stack, and it provided valuable learning experiences:
- T3 Stack Benefits: I gained a deep appreciation for the type safety and developer experience provided by the T3 stack. The combination of Next.js, TypeScript, and Prisma significantly reduced runtime errors and improved code quality.
- Database Design Importance: I learned the importance of careful database schema design, especially for applications with complex relationships like patient-doctor connections and appointment scheduling.
- Authentication Complexity: Implementing OAuth with role-based access control taught me about the nuances of secure authentication systems and session management.
- QA Process Value: Developing and following a comprehensive testing plan significantly improved the reliability of the application and reduced the number of bugs in production.
MediConnect represents an important milestone in my development journey, showcasing my ability to build complex, type-safe applications with modern web technologies. The project demonstrates my understanding of full-stack development, database design, and authentication systems.